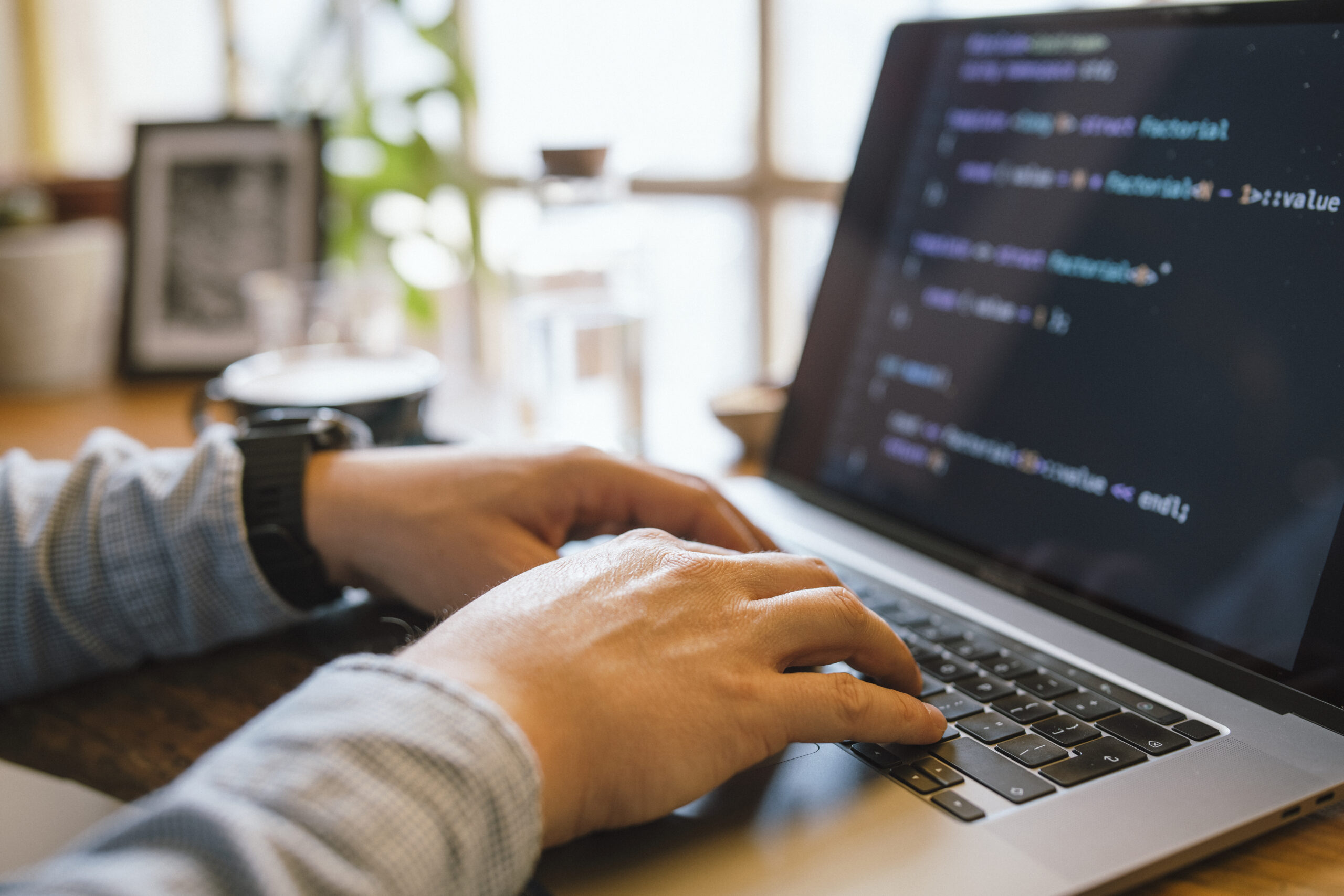
Debugging is Just about the most essential — but generally missed — abilities inside a developer’s toolkit. It is not almost repairing broken code; it’s about knowledge how and why points go Completely wrong, and learning to think methodically to unravel problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can save hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few tactics to assist developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. When composing code is a single Portion of improvement, knowing ways to communicate with it efficiently throughout execution is Similarly significant. Modern-day progress environments arrive equipped with impressive debugging abilities — but several developers only scratch the floor of what these equipment can perform.
Just take, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of accurately, they Permit you to observe exactly how your code behaves for the duration of execution, which is priceless for tracking down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective actual-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip discouraging UI issues into manageable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Variation control techniques like Git to grasp code heritage, obtain the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting to ensure when difficulties occur, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often ignored — steps in successful debugging is reproducing the issue. Prior to leaping in the code or generating guesses, developers require to produce a reliable setting or situation exactly where the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it becomes to isolate the precise circumstances less than which the bug occurs.
As you’ve collected enough information and facts, endeavor to recreate the trouble in your neighborhood setting. This may indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automated exams that replicate the sting instances or condition transitions involved. These assessments not only assistance expose the challenge but also avert regressions in the future.
Often, the issue could be environment-certain — it would materialize only on particular running systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, You may use your debugging tools more effectively, test possible fixes safely, and communicate much more clearly together with your team or customers. It turns an abstract criticism right into a concrete problem — and that’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. As opposed to viewing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications with the program. They usually tell you what precisely took place, in which it happened, and sometimes even why it took place — if you understand how to interpret them.
Start by examining the concept meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But deeper from the error stack or logs may well lie the correct root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic mistake? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some errors are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the mistake happened. Check associated log entries, enter values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the hood while not having to pause execution or action throughout the code line by line.
A superb logging approach commences with realizing what to log and at what stage. Prevalent logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for common events (like successful get started-ups), Alert for prospective concerns that don’t break the applying, ERROR for real problems, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and important determination points as part of your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging method, you may lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and correct bugs, builders will have to approach the procedure similar to a detective solving a mystery. This attitude will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the basis result in.
Start off by collecting proof. Consider the symptoms of the situation: mistake messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, acquire as much pertinent data as you could without the need of leaping to conclusions. Use logs, exam cases, and user reports to piece together a clear photograph of what’s going on.
Future, form hypotheses. Ask yourself: What could be causing this behavior? Have any alterations just lately been designed to your codebase? Has this situation transpired just before below comparable circumstances? The intention will be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the trouble inside a managed natural environment. In case you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, request your code thoughts and Permit the outcomes guide you closer to the truth.
Shell out close notice to modest particulars. Bugs generally conceal during the minimum envisioned spots—like a missing semicolon, an off-by-one mistake, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may well hide the real dilemma, just for it to resurface later.
And lastly, keep notes on That which you tried and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming concerns and enable others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed issues in sophisticated devices.
Generate Tests
Creating assessments is among the simplest tips on how to enhance your debugging expertise and Total progress performance. Checks not only aid catch bugs early but in addition serve as a safety Internet that provides you self confidence when earning variations to your codebase. A well-tested application is easier to debug because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can promptly expose no matter whether a certain piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, drastically lowering time spent debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand currently being set.
Next, combine integration assessments and finish-to-end assessments into your workflow. These aid make sure that various portions of your application work jointly easily. They’re particularly handy for catching bugs that take place in complex devices with several components or services interacting. If a thing breaks, your tests can show you which Portion of the pipeline unsuccessful and below what conditions.
Composing tests also forces you to definitely think critically regarding your code. To check a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. Once the take a look at fails consistently, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You might begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Many builders report acquiring the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support stop burnout, especially all through extended debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
For those who’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of fixing it.
Master From Every Bug
Just about every bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a thing important in the event you take some time to mirror and assess what went Erroneous.
Get started by inquiring yourself a couple of important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and enable website you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers could be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding others steer clear of the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. After all, many of the very best builders are not those who write best code, but those who continually learn from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer towards your skill established. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.